Certified Kubernetes Administration - CKA
Prerequisite
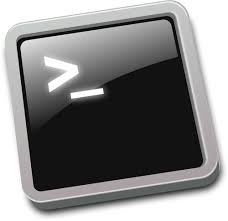
Imperative Commands
Create a pod
1 |
kubectl run --generator=run-pod/v1 nginx --image=nginx
|
Generate POD yaml file
1 |
kubectl run --generator=run-pod/v1 nginx --image=nginx --dry-run -o yaml > pod-definition.yml
|
Create Deployment
1 |
kubectl create deployment --image=nginx nginx
|
Create Deployment with replicas
1
2 |
kubectl create deployment --image=nginx nginx
kubectl scale deployment/nginx --replicas=4
|
You may want generate the deployment YAML file and than update the replica options either
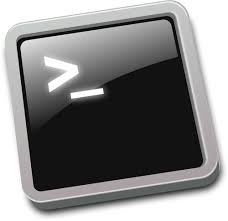
## Core Concepts
TODO
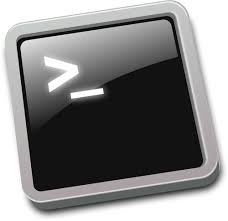
## Scheduling
TODO
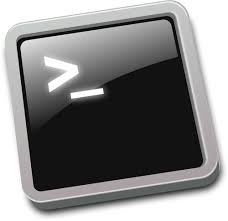
## Logging & Monitoring
1
2
3
4
5
6
7
8 |
git clone https://github.com/kodekloudhub/kubernetes-metrics-server
kubectl top node
kubectl top pod
kubectl logs -f pod-name container-name
kubectl logs -f pod-name -c TAB
|
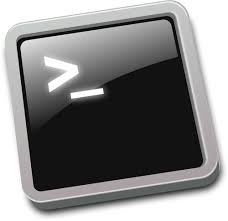
## Application Lifecycle Management
Rolling Updates and Rollbacks
- Get some commands of the first video in this theme
Recreate - Down all pods first before updating
RollingUpade - Set up/down in the same time, so the application doesn't get down
Default stategy type is rolling-update
Config and Arguments
The difference btw CMD and Entrypoint is that Entry is able to pass paremeters, like:
1
2
3 |
ENTRYPOINT sleep
docker run sleeper 10
|
And it's OK use both together to set a default parameter when it is not specified, such as:
1
2 |
ENTRYPOINT sleep
CMD ["5"] * need to pass in json format
|
Even so, you are able to change the default value, such: docker run sleeper 10
In a definition file you are to pass the parameter with spec.containers.ars: [""] options
And to overwrite the entrypoint in the definiton file use spec.contianers.command: [""]
ENTRYPOINT -> spec.contianers.command
CMD -> spec.containers.args
ConfigMaps
1
2
3
4
5
6
7
8
9
10
11
12
13
14
15
16
17
18
19
20
21
22
23
24
25
26 |
> Imperative
kubectl create configmap <config-name> --from-literal=key1=value1 --from-literal=key2=value2
> Definition file
kubectl create configmap <config-name> --from-file=configmap.yaml
cat configmap.yaml
apiVersion: v1
Kind: ConfigMap
metadata:
name: app-config
data
key1: value1
key2: value2
to set to a pod definition file
...
spec
containers:
...
envFrom:
- configMapRef:
name: app-config
There are another ways to import the data from configMap, like using volume as well
|
Secrets
1
2
3
4
5
6
7
8
9
10
11
12
13
14
15
16
17
18
19
20
21
22
23
24
25
26
27
28
29
30
31 |
> Imperative
kubectl create secret <secret-name> --from-literal=key1=value1 --from-literal=key2=value2
kubectl create secret generic db-secret --from-literal=DB_Host=sql01 --from-literal=DB_User=root --from-literal=DB_Password=password123
> Definition file
kubectl create secret <secret-name> --from-file=secret.yaml
cat secret.yaml
apiVersion: v1
Kind: Secret
metadata:
name: app-secret
data
key1: value1
key2: value2
it's desired that it data be encrypted like:
echo -n "value1" |base64
to set to a pod definition file
...
spec
containers:
...
envFrom:
- secretRef:
name: app-secret
There are another ways to import the data from configMap, like using volume as well
|
Scale Application
TODO
Multi Container
1
2
3
4
5
6
7
8
9
10
11
12 |
apiVersion: v1
kind: Pod
metadata:
name: yellow
spec:
containers:
- name: lemon
image: busybox
- name: gold
image: redis
* There are 3 common patterns in a multi-container design: side car, adapter and ambassador. It's a subject to CKAD exam and it isn't required fo CKA exam.
|
Init container
1
2
3
4
5
6
7
8
9
10
11
12
13
14
15 |
apiVersion: v1
kind: Pod
metadata:
name: myapp-pod
labels:
app: myapp
spec:
containers:
- name: myapp-container
image: busybox:1.28
command: ['sh', '-c', 'echo The app is running! && sleep 3600']
initContainers:
- name: init-myservice
image: busybox
command: ['sh', '-c', 'git clone <some-repository-that-will-be-used-by-application> ; done;']
|
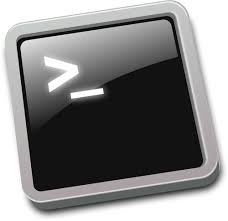
## Cluster Maintenance
1
2
3 |
kubectl drain node1 - mark the node as unscheduled and move all pod containers to another node
kubectl cordon node1 - just mark as unscheduled
kubectl uncordon node1 - mark as ready
|
Cluster upgrade
last tree minor version supported
API has to be newer
- Upgrade from v1.18.0-00 to v1.19.0-00
- on master
1
2
3
4
5
6
7
8
9
10
11
12
13
14
15 |
kubectl get nodes
kubeadm upgrade plan
kubectl drain controlplane --ignore-daemonsets
kubectl get nodes
apt update
apt install kubeadm=1.19.0-00
kubeadm upgrade plan
kubeadm upgrade apply v1.19.0
apt install kubelet=1.19.0-00
kubectl get nodes
kubectl uncordon controlplane
kubectl get nodes
kubectl drain node01 --ignore-daemonsets
ubectl taint node controlplane node-role.kubernetes.io/master-
|
- on node
1
2
3
4 |
apt update
apt install kubeadm=1.19.0-00
kubeadm upgrade node
apt install kubelet=1.19.0-00
|
Backup and restore
It's considered backups definition resource files and etcd snapshots
1
2
3
4
5
6
7
8
9
10
11
12
13
14
15
16
17
18
19
20
21
22
23
24 |
ETCDCTL_API=3 etcdctl member list --endpoints=https://127.0.0.1:2379 --cacert=/etc/kubernetes/pki/etcd/ca.crt --cert=/etc/kubernetes/pki/etcd/server.crt --key=/etc/kubernetes/pki/etcd/server.key
# backup
ETCDCTL_API=3 etcdctl --endpoints=https://127.0.0.1:2379 --cacert=/etc/kubernetes/pki/etcd/ca.crt --cert=/etc/kubernetes/pki/etcd/server.crt --key=/etc/kubernetes/pki/etcd/server.key snapshot save /opt/snapshot-pre-boot.db
# restore
* step 1:
ETCDCTL_API=3 etcdctl --endpoints=https://127.0.0.1:2379 --cacert=/etc/kubernetes/pki/etcd/ca.crt \
--cert=/etc/kubernetes/pki/etcd/server.crt --key=/etc/kubernetes/pki/etcd/server.key \
--data-dir /var/lib/etcd-from-backup \
snapshot restore /opt/snapshot-pre-boot.db
* step 2:
- change etcd definition file: /etc/kubernetes/manifests/etcd.yaml the new hostPath directory volume mount
https://github.com/mmumshad/kubernetes-the-hard-way/blob/master/practice-questions-answers/cluster-maintenance/backup-etcd/etcd-backup-and-restore.md
https://kubernetes.io/docs/tasks/administer-cluster/configure-upgrade-etcd/#backing-up-an-etcd-cluster
https://github.com/etcd-io/etcd/blob/master/Documentation/op-guide/recovery.md
https://www.youtube.com/watch?v=qRPNuT080Hk
|
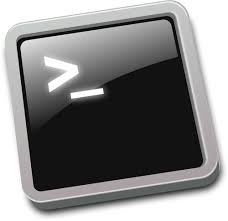
## Security
TODO
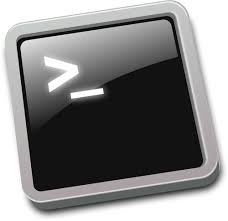
## Storage